Arduino Nano Control Servo Motor via Bluetooth
This tutorial instructs you how to program an Arduino Nano to manage a Servo Motor using either Bluetooth (HC-05 module) or BLE (HM-10 module). Instructions for both modules will be provided.
We will use the Bluetooth Serial Monitor App on a smartphone to transmit the angle value to Arduino Nano. Arduino Nano will adjust the servo motor in accordance with the received value.
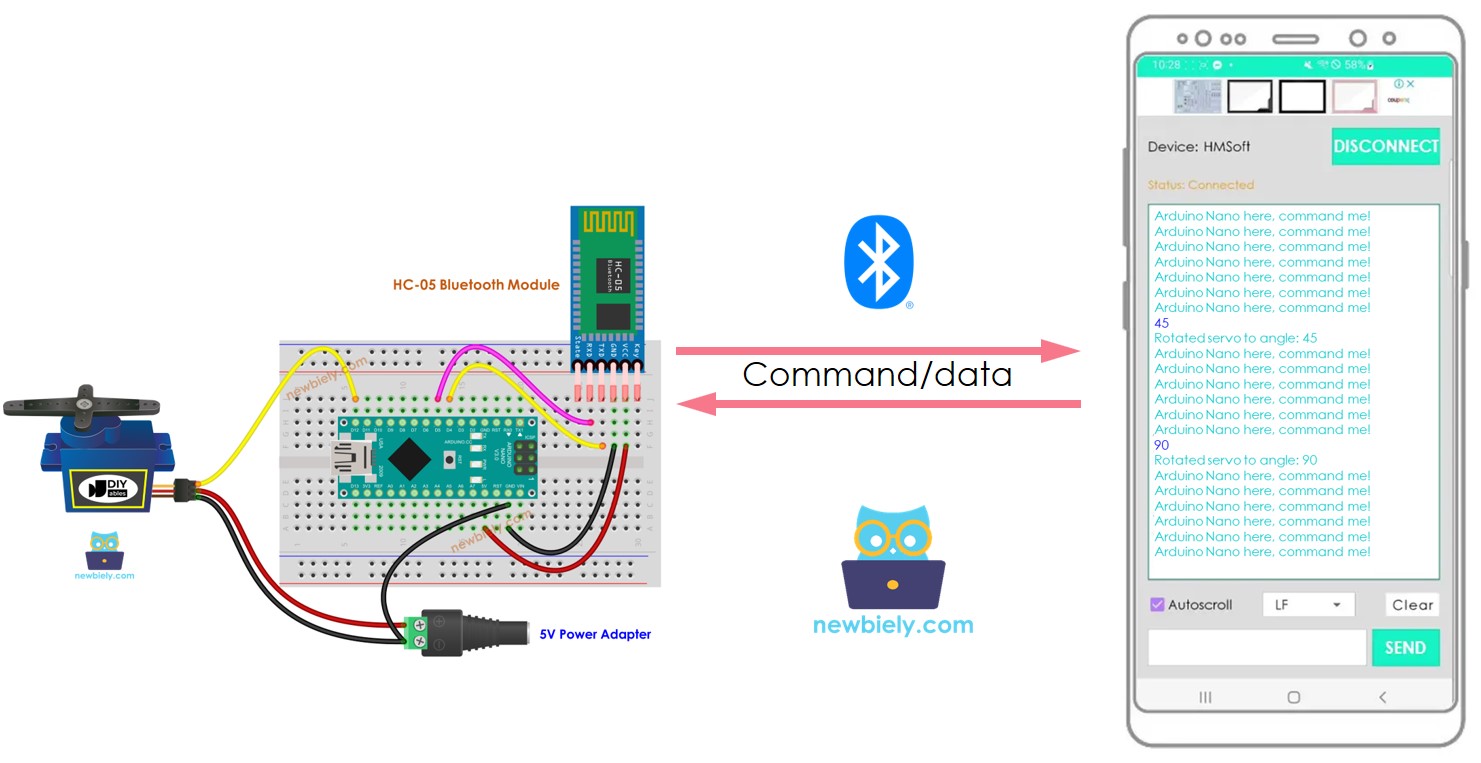
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Servo Motor and Bluetooth Module
If you are not familiar with Servo Motors, Bluetooth Modules, their pinouts, how they work, and how to program them, please refer to the following tutorials for more information:
Wiring Diagram
- To manage a Servo Motor with Classic Bluetooth, the HC-05 Bluetooth module should be used and the wiring diagram provided should be consulted.
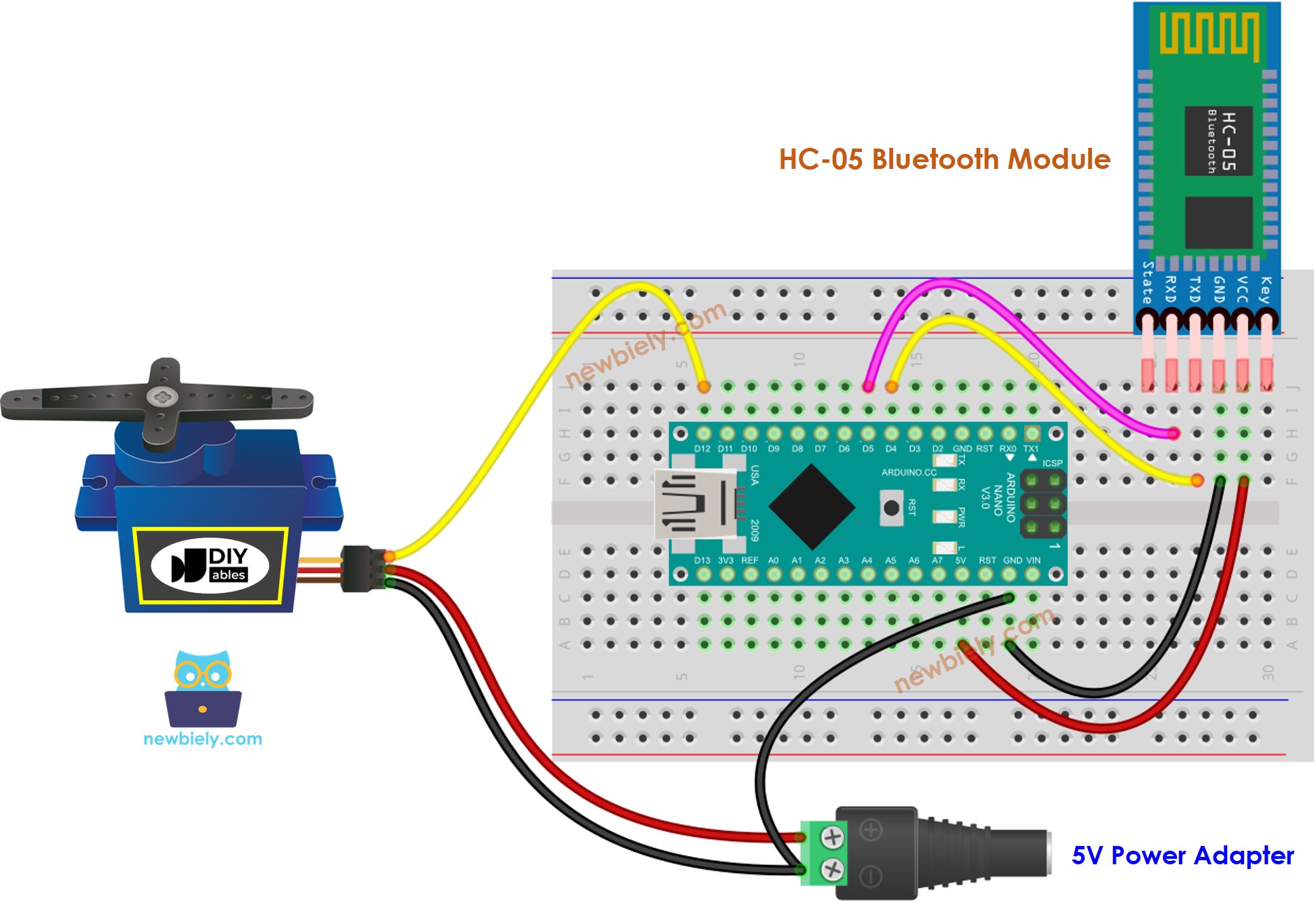
This image is created using Fritzing. Click to enlarge image
- To operate a Servo Motor with BLE, the HM-10 BLE module should be used. The wiring diagram for this is given below.
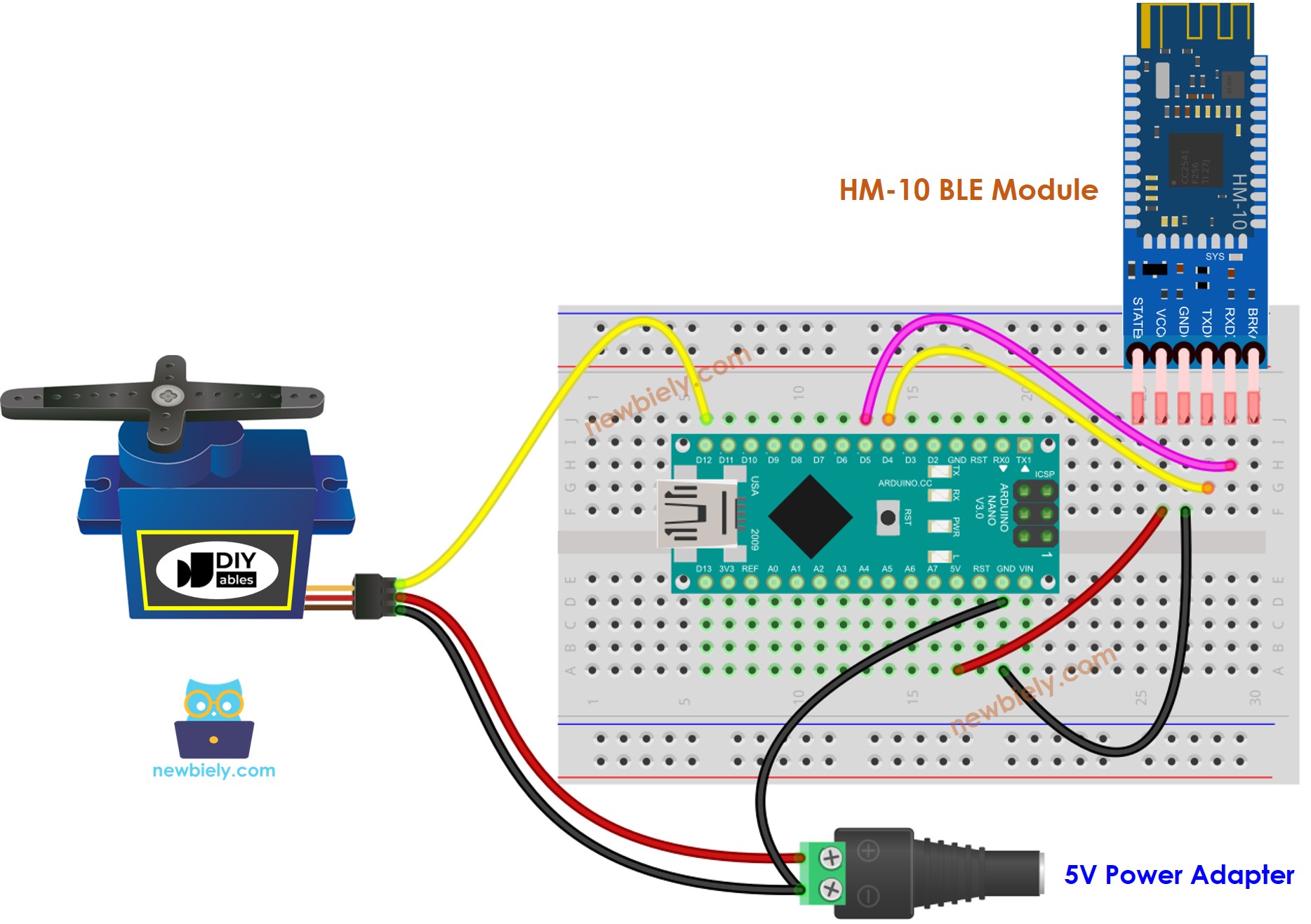
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code - controls Servo Motor via Bluetooth/BLE
The code given here is able to be used with both the HC-10 Bluetooth module and the HM-10 BLE module.
Detailed Instructions
- Install Bluetooth Serial Monitor App on your smartphone.
- Take the code given and open it in the Arduino IDE, then upload it to your Arduino Nano board.
- In case you have difficulty uploading the code, try disconnecting the TX and RX pins from the Bluetooth module, then upload the code, and afterwards reconnect the RX/TX pins.
- Open the Bluetooth Serial Monitor App on your smartphone and choose either Classic Bluetooth or BLE, depending on the module you are using.
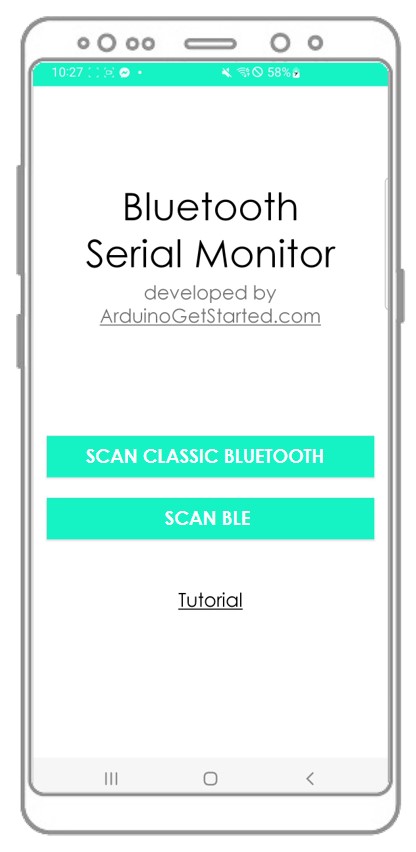
- Connect the app to the HC-05 Bluetooth module or HM-10 BLE module.
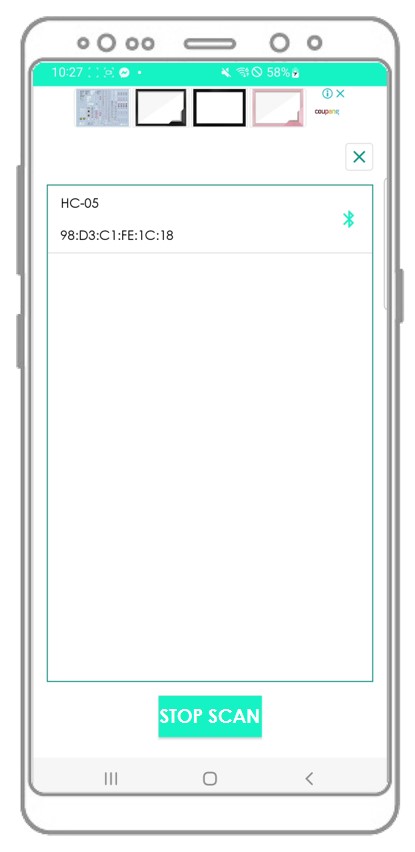
- Enter an angle such as 45 or 90 and press the Send button.
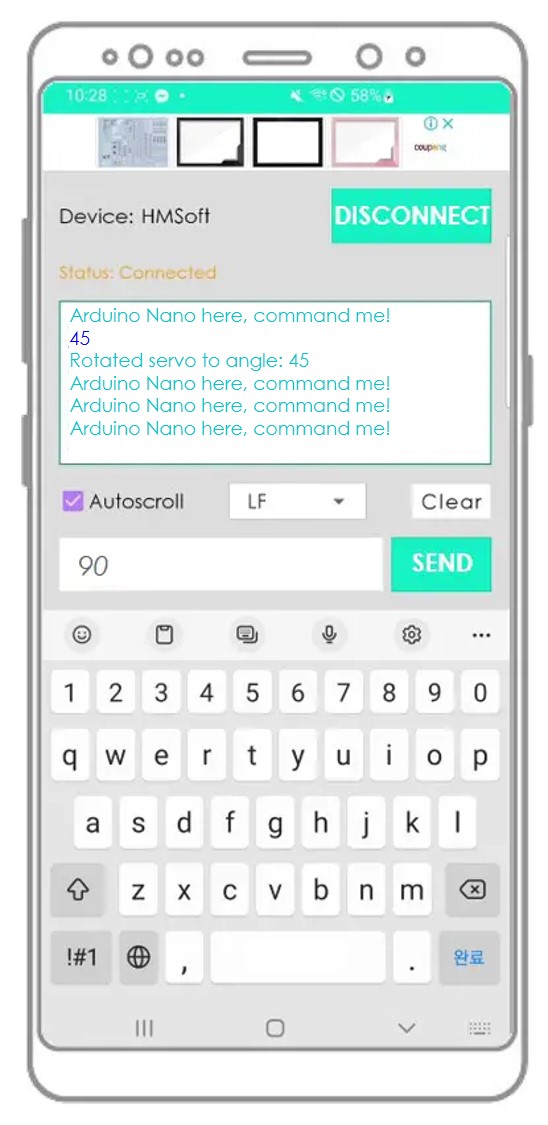
- Witness the Servo Motor's angle alteration.
- Examine the outcomes on the Android App.
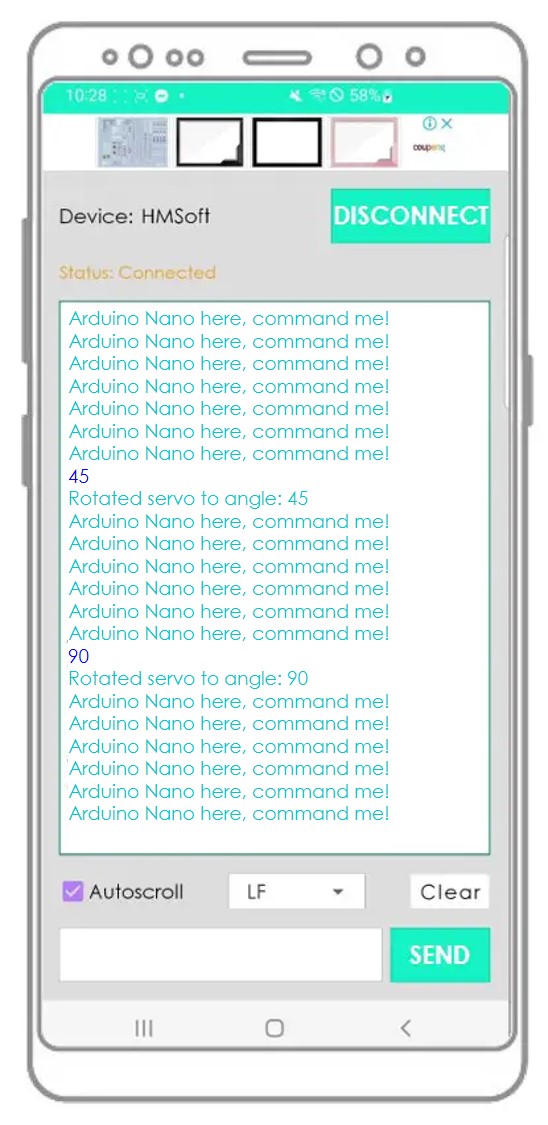
If you find the Bluetooth Serial Monitor app helpful, please rate it 5 stars on Play Store. Thank you for your support!